Autoencoders
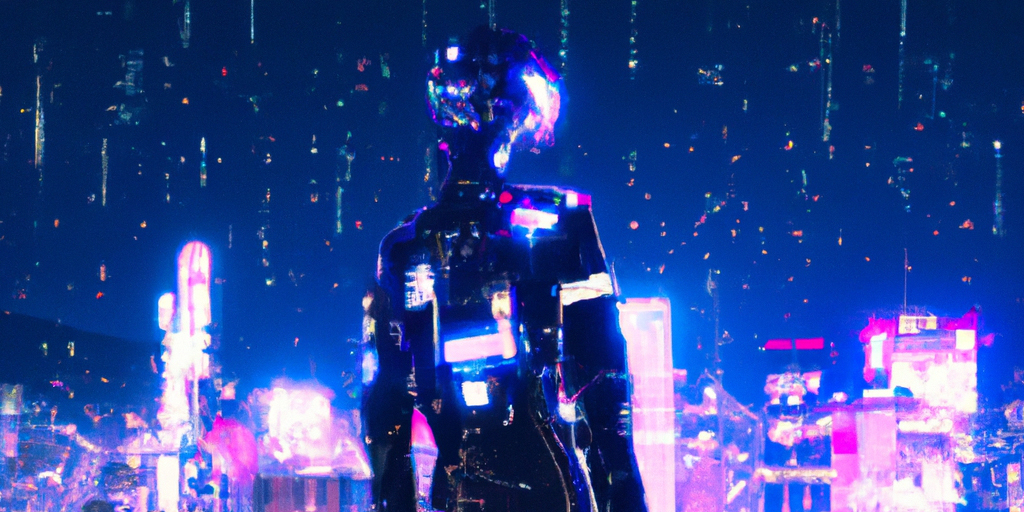
An autoencoder is a type of neural network used to learn an input’s compressed representation. It is composed of two parts: an encoder and a decoder. The encoder takes in an input and converts it into a hidden representation, or encoding, which is typically smaller in size than the input. The decoder then converts this encoding back into an output similar to the original input. Autoencoders are often used for dimensionality reduction or to learn a compact representation of input data, which can then be used for other tasks such as data visualization or data denoising.
Here is an example of how you can use autoencoders to compress and reconstruct images from the MNIST dataset in Python using the Keras library:
from keras.layers import Input, Dense from keras.models import Model # Load and preprocess the MNIST data (x_train, y_train), (x_test, y_test) = mnist.load_data() x_train = x_train.astype('float32') / 255. x_test = x_test.astype('float32') / 255. x_train = x_train.reshape((len(x_train), np.prod(x_train.shape[1:]))) x_test = x_test.reshape((len(x_test), np.prod(x_test.shape[1:]))) # Define the autoencoder model input_dim = x_train.shape[1] encoding_dim = 32 input_img = Input(shape=(input_dim,)) encoded = Dense(encoding_dim, activation='relu')(input_img) decoded = Dense(input_dim, activation='sigmoid')(encoded) autoencoder = Model(input_img, decoded) # Compile and fit the model autoencoder.compile(optimizer='adam', loss='binary_crossentropy') autoencoder.fit(x_train, x_train, epochs=50, batch_size=256, shuffle=True, validation_data=(x_test, x_test)) # Use the autoencoder to compress and reconstruct the images encoder = Model(input_img, encoded) encoded_imgs = encoder.predict(x_test) decoded_imgs = autoencoder.predict(x_test)
This code defines and trains an autoencoder with a single hidden layer that has 32 units. The autoencoder is trained by minimizing the binary cross-entropy loss using the Adam optimizer. After training, the encoder can be used to compress the images by mapping them to the latent space, and the decoder can be used to reconstruct the images from the latent representation.