Reinforcement learning and how it works
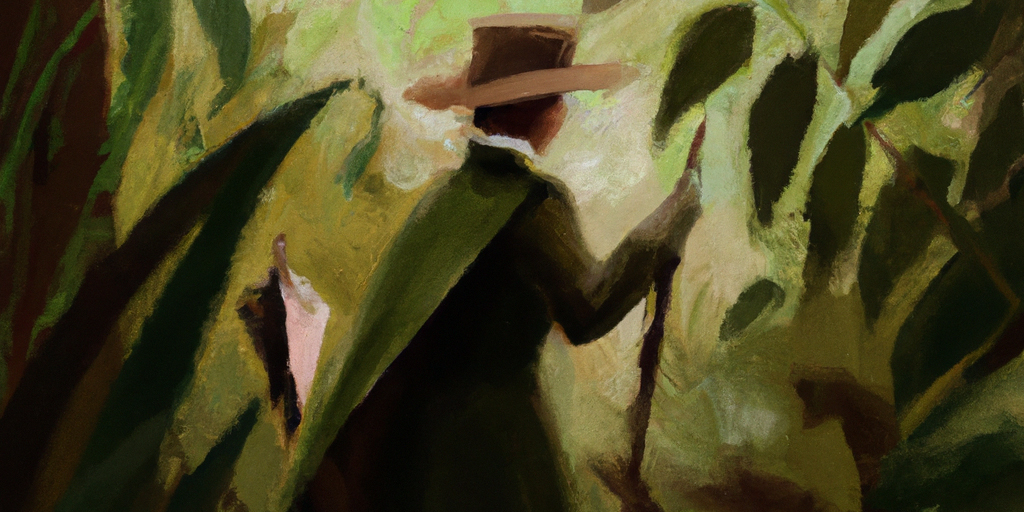
Reinforcement learning is a type of machine learning that involves training an agent to make a series of decisions in an environment to maximize a reward. The agent learns through trial and error by taking actions and receiving feedback through rewards or penalties.
Here’s how it works:
- The agent receives the current state of the environment as input.
- The agent chooses an action based on that input and its current state of knowledge.
- The environment responds to the action by transitioning to a new state and returning an observation and reward to the agent.
- The agent uses this feedback to update its internal model of the environment and the value of the actions it can take.
- The process repeats, with the agent continually adjusting its actions based on the rewards it receives.
Over time, the agent learns to take actions that maximize the reward, and it becomes better at achieving its goals in the environment.
Here is a simple example of reinforcement learning in Python using the OpenAI Gym library:
import gym # Load the CartPole-v0 environment env = gym.make('CartPole-v0') # Set the number of actions n_actions = env.action_space.n # Set the number of observations n_observations = env.observation_space.shape[0] # Set the maximum number of steps per episode max_steps_per_episode = 1000 # Set the learning rate learning_rate = 0.01 # Set the discount rate discount_rate = 0.99 # Set the exploration rate exploration_rate = 1.0 exploration_decay_rate = 0.001 # Initialize the weights weights = [0.0] * n_actions # Initialize the bias bias = 0.0 # Initialize the Q-table q_table = [] # Set the number of episodes n_episodes = 1000 # Train the agent for episode in range(n_episodes): # Reset the environment obs = env.reset() # Initialize the cumulative reward cum_reward = 0 # Initialize the steps steps = 0 # Run the episode while True: # Choose an action action = 0 if np.matmul(weights, obs) + bias < 0 else 1 # Take the action next_obs, reward, done, _ = env.step(action) # Update the Q-value best_q = max(q_table[next_obs]) q_table[obs][action] = (1 - learning_rate) * q_table[obs][action] + learning_rate * (reward + discount_rate * best_q) # Update the cumulative reward cum_reward += reward # Update the current observation obs = next_obs # Check if the episode is over if done: break # Print the cumulative reward for the episode print(f'Episode {episode}: {cum_reward}')
This is just a simple example to illustrate the basic concepts of reinforcement learning. In practice, you may want to use more advanced techniques, such as a Q-learning algorithm or a neural network, to train your agent.